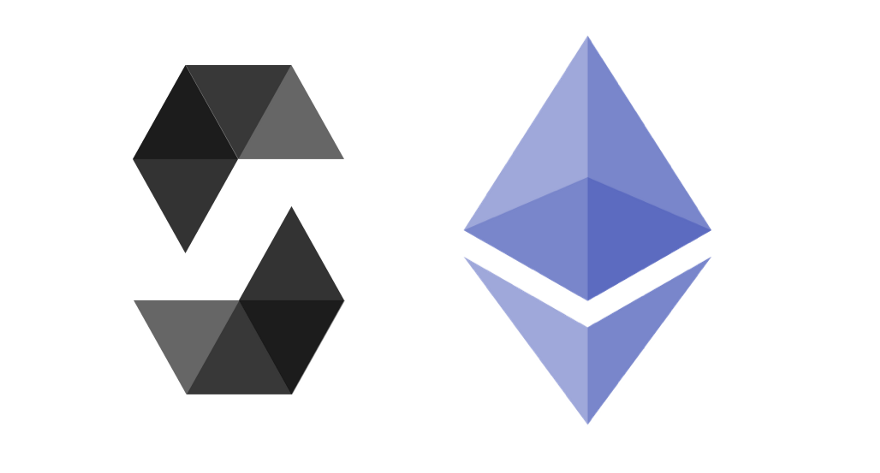
In Ethereum smart contracts, the Function Selector is a 4-byte identifier used to identify a specific function. It is calculated by hashing the function signature with Keccak-256 and taking the first 4 bytes of the result.
Calculation Method
- The function signature is a combination of the function name and its parameter types, e.g., transfer(address,uint256).
- Apply Keccak-256 hashing to the function signature.
- Take the first 4 bytes of the hash result.
Example
Function signature:
transfer(address,uint256)
Steps to calculate:
1.Hash of the function signature:
keccak256("transfer(address,uint256)") = 0xa9059cbb…
2.The first 4 bytes are the function selector:
0xa9059cbb
This selector is encoded in the first 4 bytes of the transaction data when calling a contract, allowing the contract to identify which specific function to execute.
Function Selector in Solidity
In Solidity, you can obtain a function selector using abi.encodeWithSelector or this.function.selector.
Example Code
pragma solidity ^0.8.0;
contract SelectorExample {
function transfer(address to, uint256 value) public pure returns (bytes4) {
return this.transfer.selector; // Returns the function selector 0xa9059cbb
}
}
Practical Applications
- Contract ABI Encoding: Function selectors are part of the data used to call a contract.
- Low-Level Calls: Used in low-level interfaces like call to pass the function selector.
- Function Overloading: Different function signatures generate different selectors, enabling function overloading.
- Security: Selector collisions can lead to vulnerabilities, so developers should avoid potential collisions when designing functions.
Example: Low-Level Call
pragma solidity ^0.8.0;
contract CallExample {
function callTransfer(address contractAddress, address to, uint256 amount) external {
bytes4 selector = bytes4(keccak256("transfer(address,uint256)"));
(bool success, ) = contractAddress.call(
abi.encodeWithSelector(selector, to, amount)
);
require(success, "Call failed");
}
}
This approach is often used to dynamically generate call data or call functions via proxy contracts.